Java 17 migration tips/checklist
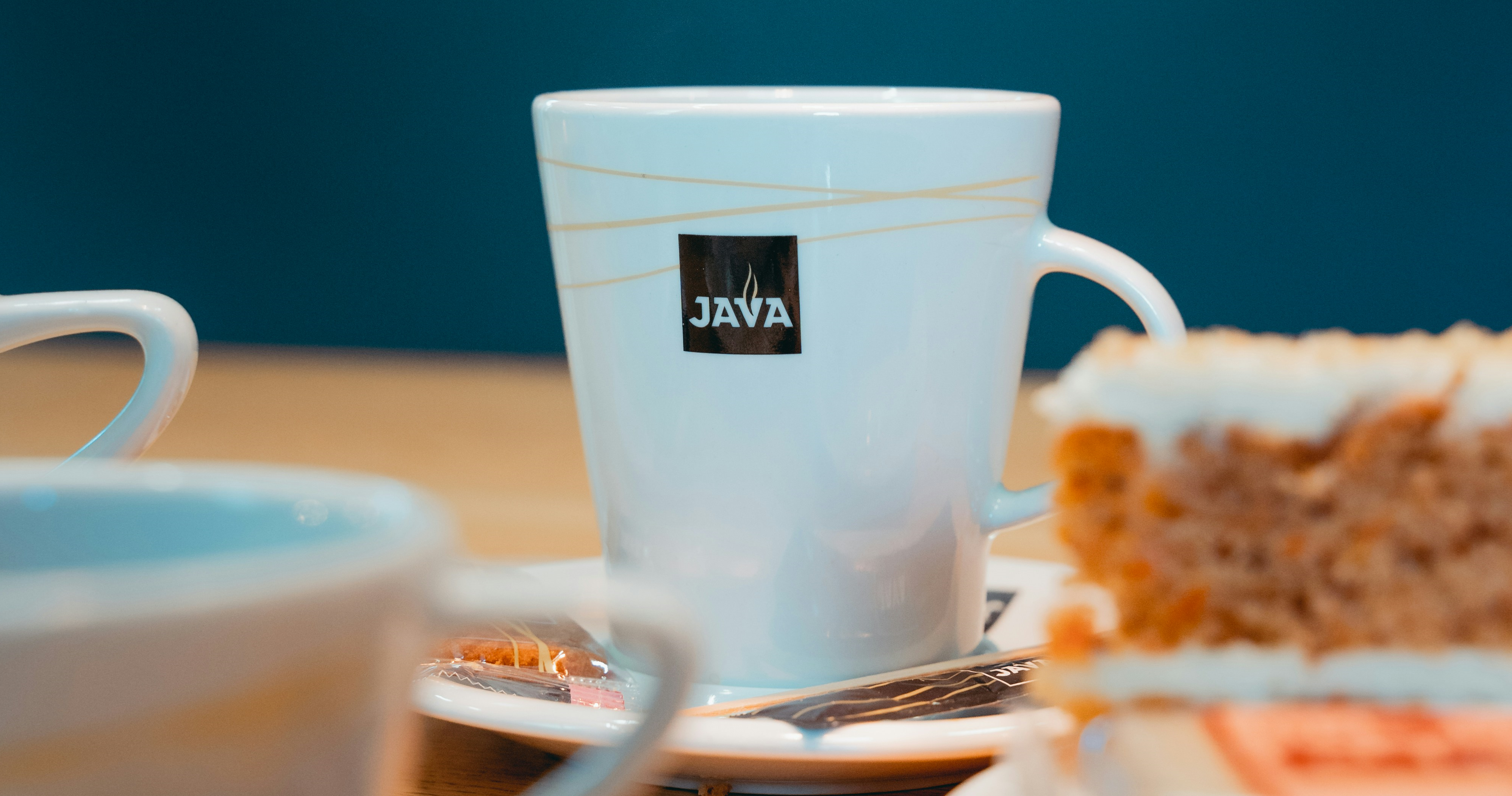
Introduction
Navigating the Java 17, Spring 6, and Spring Boot 3 Upgrade Journey can be a challenging yet rewarding endeavor. As you embark on this journey, it’s crucial to understand the scope of the upgrade and the key considerations involved in each component’s migration. In this article, we’ll delve into essential aspects of upgrading, including migrating from Java EE to Jakarta EE in Spring 6, updating Hibernate configurations for Java 17 and Spring 6, upgrading API documentation from Swagger to OpenAPI, transitioning to Apache HttpClient 5, and migrating to Java 17 with Spring Boot 3 using OpenRewrite.
Migrating from Java EE to Jakarta EE in Spring 6
When upgrading to Spring 6 and Spring Boot 3, compatibility with Java EE or Jakarta EE APIs is paramount. This entails updating imports and configurations to align with namespace changes. For instance, understanding the mapping between Java EE and Jakarta EE namespaces is crucial for a seamless migration:
Java EE Namespace | Jakarta EE Namespace |
---|---|
javax.servlet | jakarta.servlet |
javax.servlet.http | jakarta.servlet.http |
javax.servlet.annotation | jakarta.servlet.annotation |
javax.servlet.descriptor | jakarta.servlet.descriptor |
javax.servlet.jsp | jakarta.servlet.jsp |
javax.servlet.jsp.el | jakarta.servlet.jsp.el |
javax.servlet.jsp.tagext | jakarta.servlet.jsp.tagext |
javax.websocket | jakarta.websocket |
javax.websocket.server | jakarta.websocket.server |
javax.xml.* | jakarta.xml.* |
javax.activation | jakarta.activation |
javax.annotation | jakarta.annotation |
javax.enterprise | jakarta.enterprise |
javax.jms | jakarta.jms |
javax.jws | jakarta.jws |
javax.mail | jakarta.mail |
javax.management | jakarta.management |
javax.persistence | jakarta.persistence |
javax.security.* | jakarta.security.* |
javax.transaction | jakarta.transaction |
javax.validation | jakarta.validation |
javax.websocket | jakarta.websocket |
javax.xml.* | jakarta.xml.* |
Hibernate Update in Java 17 and Spring 6
Upgrading to Java 17 and Spring 6 necessitates considerations for updating Hibernate configurations. Key points to note include updating the Hibernate dialect and annotations for defining custom types. Ensuring compatibility with the latest Hibernate versions and adhering to evolving best practices is essential.
Hibernate Dialect Update: The
org.hibernate.dialect.MySQLDialect
is supported from Hibernate 5.3 onwards. Prior to Hibernate 5.3, it was recommended to useorg.hibernate.dialect.MySQL57Dialect
for MySQL 5.x and 8.x. However, with the release of Hibernate 5.3, aorg.hibernate.dialect.MySQL8Dialect
was introduced, and it is recommended to useorg.hibernate.dialect.MySQLDialect
sinceHibernate 6
, asorg.hibernate.dialect.MySQL8Dialect
is deprecated.Type and TypeDef Annotations Update: In Hibernate 6, there are changes in the annotations used for defining custom types. For example, the usage of
@TypeDef
and@Type
annotations has been updated.
|
|
Instead of:
|
|
You should now use:
|
|
API documentation upgrade swagger to OpenAPI
Migrating from Swagger annotations to OpenAPI annotations involves updating your codebase to use the newer annotations provided by the springdoc-openapi
library, as well as adjusting any configuration or usage accordingly. Here’s a list of some common changes you may need to make:
Swagger Annotation | OpenAPI (springdoc-openapi) Annotation |
---|---|
io.swagger.annotations.ApiIgnore | org.springdoc.core.annotations.Hidden |
io.swagger.annotations.ApiModelProperty | io.swagger.v3.oas.annotations.media.Schema |
io.swagger.annotations.ApiParam | io.swagger.v3.oas.annotations.Parameter |
io.swagger.annotations.ApiOperation | io.swagger.v3.oas.annotations.Operation |
io.swagger.annotations.ApiResponse | io.swagger.v3.oas.annotations.responses.ApiResponse |
io.swagger.annotations.ApiModel | io.swagger.v3.oas.annotations.media.Schema |
io.swagger.annotations.ApiImplicitParams | io.swagger.v3.oas.annotations.parameters.Parameters |
io.swagger.annotations.ApiResponses | io.swagger.v3.oas.annotations.responses.ApiResponse |
Swagger Annotation: @ApiIgnore
|
|
OpenAPI (springdoc-openapi) Annotation: @Hidden
|
|
Swagger Annotation: @ApiModelProperty
|
|
OpenAPI (springdoc-openapi) Annotation: @Schema
|
|
Upgrading to Apache HttpClient 5
Key Differences:
Package Names
: HttpClient 5 usesorg.apache.hc.client5.http
and related packages, whereas HttpClient 4 usesorg.apache.http.
Class Structure
: HttpClient 5 introduces new classes and restructures existing ones for configuration and connection management.SSL Context Handling
: HttpClient 5 provides a different approach for SSL context handling usingSSLConnectionSocketFactoryBuilder
.Connection Pooling Configuration
: Configuration related to connection pooling is done differently inHttpClient 5
withPoolingHttpClientConnectionManagerBuilder
.Proxy Configuration
: HttpClient 5 proxy configuration involves changes in howHttpHost
is used.
New updated migrated code
Additional Notes:
- WebClient: Remember that WebClient is the recommended alternative to RestTemplate for new applications, as it provides both synchronous and asynchronous capabilities.
- RestTemplate: However, if you’re working with existing code, RestTemplate remains a viable choice.
The new webclient will be
Migrating to Java 17 with Spring Boot 3 using OpenRewrite
Introduction to OpenRewrite
OpenRewrite is a powerful tool for automating code refactoring and transformation tasks in Java projects. It provides a flexible framework for writing custom rules to analyze and modify Java code programmatically. In this section, we’ll explore how to integrate OpenRewrite into both Maven and Gradle projects to facilitate the migration from Java 11 to Java 17, along with Spring Boot 3.
|
|
|
|
Integrating OpenRewrite into Maven Projects
Step 1: Add OpenRewrite Plugin to pom.xml
To use OpenRewrite in a Maven project, first, add the OpenRewrite Maven plugin to your project’s pom.xml
:
|
|
Run mvn rewrite:run
to run the recipe.
alternatively
|
|
Integrating OpenRewrite into Gradle Projects
Step 1: Add OpenRewrite Plugin to gradle
- Add the following to your
build.gradle
file:
|
|
- Run
gradle rewriteRun
to run the recipe.